Lab 18
Lab18: Car objects
Introduction
The goal for this activity is to implement a Car
class so that it conforms to the UML below:
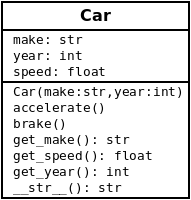
Part 1: Driver, Constructor and __str__
- Create a Python file car.py.
- Add the three instance variables from the UML diagram to your
Car
class. - Code the constructor(__init__) for your
Car
class. This should intialize themake
andyear
fields using the arguments to the constructor. Thespeed
should be initialized to 0. - Code the
__str__
method. This method should take no parameters and should return a string generated using theformat
method for strings:str_car = "A {} {} that is going {%.1f} mph"
str_car.format(year, make, speed)
- Test your partially completed
Car
class by adding code to amain
section in car.py that creates and prints two car objects. The contents ofmain
should look something like the following.car1 = Car("Ford", 1997) car2 = Car("Toyota", 2014) print(car1) print(car2)
Part 2: Remaining Methods
Complete each of the remaining methods of the Car
class. Test each method by adding appropriate calls to main
.
- The three "getter" methods should each return the value of the appropriate instance variable.
- The
accelerate
method should increase the current speed of the car by 5.0 mph. It should NOT be possible to increase the speed beyond 150.0 mph. If a call toaccelerate
would push the speed beyond 150.0, the speed should be set to 150.0.
(How will you test that this is working correctly? How many times do you need to callaccelerate
before the upper speed limit is reached? Might a loop be helpful here?) - The
brake
method should decrease the current speed by 5.0 mph. It should not be possible for the speed to drop below 0 mph.
Submit car.py through Gradescope by the end of the day.
Acknowledgements
This references a Java lab designed by Dr. Sprague.